The MERN stack (MongoDB, Express.js, React, Node.js) is a powerful framework for building modern web applications. However, as applications grow, so do the challenges of maintaining speed, scalability, and efficiency. Optimizing your MERN stack app is crucial to delivering a seamless user experience. Here are the top 5 best practices to ensure your MERN applications perform optimally.
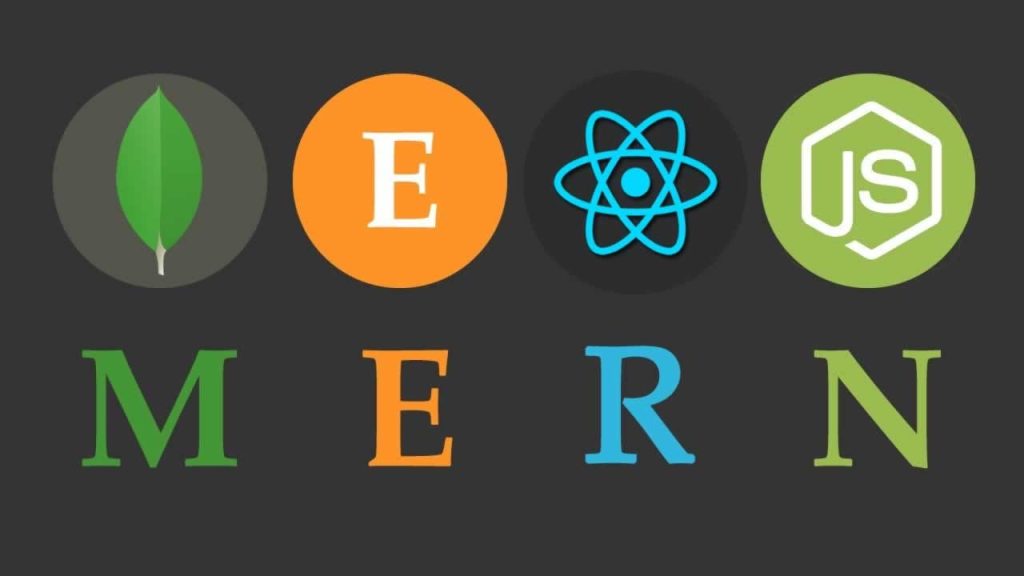
1. Optimize Database Queries with MongoDB
MongoDB is a NoSQL database that provides flexibility, but poorly structured queries can lead to significant performance bottlenecks.
Best Practices:
- Use Indexes: Proper indexing can dramatically reduce query execution time. Index frequently queried fields to speed up data retrieval.
- Avoid Unnecessary Data Fetching: Only fetch the fields you need by using projections. For example, avoid retrieving entire documents if you only need a few fields.
- Limit Document Size: MongoDB documents can be large, which can slow down operations. Avoid embedding large amounts of data in a single document. Instead, consider breaking it into smaller sub-documents or related collections.
2. Use Lazy Loading and Code Splitting in React
React is an essential part of the MERN stack, but as your app grows, so does the bundle size, leading to slow loading times. Implementing lazy loading and code splitting can drastically reduce initial load times.
Best Practices:
- Lazy Loading Components: Instead of loading everything at once, load components only when needed. This reduces the size of the initial JavaScript bundle.
- Code Splitting: Use tools like Webpack to split your app into smaller chunks that are loaded as needed.
3. Implement Caching for Faster Response Times
Caching is one of the most effective ways to enhance the performance of your MERN stack applications, particularly for frequently accessed data. Caching can be implemented on both the client and server sides.
Best Practices:
- Use Redis or Memcached: These in-memory data stores can cache database queries, reducing the need to hit the database on every request.
- HTTP Caching: Cache static assets like images, CSS, and JavaScript files using browser caching techniques to minimize server load.
- Client-Side Caching: Implement caching mechanisms in React using service workers or libraries like React Query to store frequently accessed API data.
4. Leverage Asynchronous Operations in Node.js
Node.js is inherently asynchronous, and using its non-blocking nature effectively can significantly improve the performance of your backend. Blocking operations can reduce throughput and slow down your server.
Best Practices:
- Async/Await: Always use async/await to manage asynchronous operations, ensuring that your Node.js server can handle multiple requests simultaneously without being bogged down by one task.
- Use Streams: For large data transfers, such as sending files, using streams can help you send chunks of data instead of loading everything into memory at once.
5. Monitor and Optimize API Performance
A well-structured API is essential for a performant MERN stack application. Monitoring and optimizing your APIs can help ensure they handle large volumes of traffic and data efficiently.
Best Practices:
- Paginate Responses: For large datasets, don’t return everything in one response. Use pagination to break data into smaller, manageable chunks.
- Limit Payload Size: Avoid sending large JSON payloads in API responses. Minimize the data sent by only including essential fields.
- Rate Limiting: Prevent abuse and overload by implementing rate limiting to control the number of requests a client can make in a given period.
Conclusion
Optimizing a MERN stack application requires a combination of database query efficiency, front-end code management, and server-side performance improvements. By following these best practices—optimizing MongoDB queries, leveraging lazy loading and code splitting in React, implementing caching, using async operations in Node.js, and monitoring API performance—you can ensure your application scales well, delivers fast load times, and provides a seamless experience for your users.
Investing in performance optimization from the beginning will not only improve user satisfaction but also reduce the load on your infrastructure as your application grows.